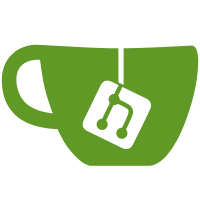
Added has-changed detection flag Fixed dialog result null-value Changed session manager event names
186 lines
7.4 KiB
C#
186 lines
7.4 KiB
C#
using System.Windows.Forms;
|
|
using FizzyLauncher.Models;
|
|
using RyzStudio.Windows.Forms;
|
|
using RyzStudio.Windows.ThemedForms;
|
|
using RyzStudio.Windows.ThemedForms.ButtonTextBox;
|
|
using RyzStudio.Windows.ThemedForms.PickerBox;
|
|
|
|
namespace FizzyLauncher
|
|
{
|
|
public class EditGroupForm : TDialog
|
|
{
|
|
private Label label2;
|
|
private Label label1;
|
|
private ThButton button1;
|
|
private ThYesNoPickerBox pickerBox1;
|
|
private THorizontalSeparator horizontalSeparator2;
|
|
private ThClearableTextBox textBox1;
|
|
|
|
private TileGroupModel result = null;
|
|
|
|
|
|
public EditGroupForm(TileGroupModel model = null)
|
|
{
|
|
InitializeComponent();
|
|
|
|
result = model;
|
|
|
|
StartPosition = FormStartPosition.WindowsDefaultLocation;
|
|
Text = result == null ? "Add Group" : "Edit Group";
|
|
OkButton = button1;
|
|
|
|
pickerBox1.ComboBox.Items.Clear();
|
|
pickerBox1.ComboBox.Items.AddRange(new string[] { "No", "Yes" });
|
|
if (pickerBox1.ComboBox.Items.Count > 0)
|
|
{
|
|
pickerBox1.ComboBox.SelectedIndex = 0;
|
|
}
|
|
|
|
if (result != null)
|
|
{
|
|
textBox1.Text = result.Title?.Trim() ?? string.Empty;
|
|
pickerBox1.Value = result.IsExpanded;
|
|
}
|
|
}
|
|
|
|
private void InitializeComponent()
|
|
{
|
|
System.ComponentModel.ComponentResourceManager resources = new System.ComponentModel.ComponentResourceManager(typeof(EditGroupForm));
|
|
textBox1 = new ThClearableTextBox();
|
|
label2 = new Label();
|
|
label1 = new Label();
|
|
button1 = new ThButton();
|
|
pickerBox1 = new ThYesNoPickerBox();
|
|
horizontalSeparator2 = new THorizontalSeparator();
|
|
SuspendLayout();
|
|
//
|
|
// textBox1
|
|
//
|
|
textBox1.AcceptButton = null;
|
|
textBox1.Anchor = AnchorStyles.Top | AnchorStyles.Left | AnchorStyles.Right;
|
|
textBox1.BackColor = System.Drawing.Color.Transparent;
|
|
textBox1.ClearedValue = "";
|
|
textBox1.EnableReactiveVisual = true;
|
|
textBox1.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F);
|
|
textBox1.HighlightImage = (System.Drawing.Image)resources.GetObject("textBox1.HighlightImage");
|
|
textBox1.Location = new System.Drawing.Point(192, 20);
|
|
textBox1.Margin = new Padding(10, 10, 10, 0);
|
|
textBox1.Name = "textBox1";
|
|
textBox1.NormalImage = (System.Drawing.Image)resources.GetObject("textBox1.NormalImage");
|
|
textBox1.Padding = new Padding(4, 4, 3, 3);
|
|
textBox1.Size = new System.Drawing.Size(177, 32);
|
|
textBox1.TabIndex = 152;
|
|
textBox1.TabStop = false;
|
|
textBox1.UseSystemPasswordChar = false;
|
|
//
|
|
// label2
|
|
//
|
|
label2.AutoSize = true;
|
|
label2.BackColor = System.Drawing.Color.Transparent;
|
|
label2.ForeColor = System.Drawing.SystemColors.ControlText;
|
|
label2.Location = new System.Drawing.Point(10, 62);
|
|
label2.Name = "label2";
|
|
label2.Padding = new Padding(0, 9, 0, 10);
|
|
label2.Size = new System.Drawing.Size(91, 34);
|
|
label2.TabIndex = 155;
|
|
label2.Text = "Show Expanded";
|
|
label2.TextAlign = System.Drawing.ContentAlignment.MiddleLeft;
|
|
//
|
|
// label1
|
|
//
|
|
label1.AutoSize = true;
|
|
label1.BackColor = System.Drawing.Color.Transparent;
|
|
label1.ForeColor = System.Drawing.SystemColors.ControlText;
|
|
label1.Location = new System.Drawing.Point(10, 21);
|
|
label1.Margin = new Padding(0);
|
|
label1.Name = "label1";
|
|
label1.Padding = new Padding(0, 9, 0, 10);
|
|
label1.Size = new System.Drawing.Size(29, 34);
|
|
label1.TabIndex = 153;
|
|
label1.Text = "Title";
|
|
label1.TextAlign = System.Drawing.ContentAlignment.MiddleLeft;
|
|
//
|
|
// button1
|
|
//
|
|
button1.AcceptButton = null;
|
|
button1.ActiveImage = null;
|
|
button1.Anchor = AnchorStyles.Bottom | AnchorStyles.Right;
|
|
button1.BackColor = System.Drawing.Color.Transparent;
|
|
button1.EnableMenuOnClick = false;
|
|
button1.EnableReactiveVisual = true;
|
|
button1.HoverImage = null;
|
|
button1.IdleImage = null;
|
|
button1.LabelText = "&Save";
|
|
button1.Location = new System.Drawing.Point(241, 469);
|
|
button1.Margin = new Padding(10, 10, 10, 0);
|
|
button1.Name = "button1";
|
|
button1.Padding = new Padding(4, 4, 3, 3);
|
|
button1.Size = new System.Drawing.Size(128, 32);
|
|
button1.TabIndex = 173;
|
|
button1.TabStop = false;
|
|
//
|
|
// pickerBox1
|
|
//
|
|
pickerBox1.AcceptButton = null;
|
|
pickerBox1.Anchor = AnchorStyles.Top | AnchorStyles.Right;
|
|
pickerBox1.BackColor = System.Drawing.Color.Transparent;
|
|
pickerBox1.EnableReactiveVisual = true;
|
|
pickerBox1.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F);
|
|
pickerBox1.Location = new System.Drawing.Point(285, 62);
|
|
pickerBox1.Name = "pickerBox1";
|
|
pickerBox1.Padding = new Padding(4, 4, 3, 3);
|
|
pickerBox1.SelectedIndex = 0;
|
|
pickerBox1.Size = new System.Drawing.Size(84, 34);
|
|
pickerBox1.TabIndex = 174;
|
|
pickerBox1.TabStop = false;
|
|
pickerBox1.Value = false;
|
|
//
|
|
// horizontalSeparator2
|
|
//
|
|
horizontalSeparator2.Anchor = AnchorStyles.Bottom | AnchorStyles.Left | AnchorStyles.Right;
|
|
horizontalSeparator2.AutoScrollMargin = new System.Drawing.Size(0, 0);
|
|
horizontalSeparator2.AutoScrollMinSize = new System.Drawing.Size(0, 0);
|
|
horizontalSeparator2.BackColor = System.Drawing.Color.Transparent;
|
|
horizontalSeparator2.Location = new System.Drawing.Point(10, 437);
|
|
horizontalSeparator2.Margin = new Padding(0, 10, 0, 0);
|
|
horizontalSeparator2.MaximumSize = new System.Drawing.Size(4920, 2);
|
|
horizontalSeparator2.MinimumSize = new System.Drawing.Size(0, 22);
|
|
horizontalSeparator2.Name = "horizontalSeparator2";
|
|
horizontalSeparator2.Size = new System.Drawing.Size(364, 22);
|
|
horizontalSeparator2.TabIndex = 182;
|
|
horizontalSeparator2.TabStop = false;
|
|
//
|
|
// EditGroupForm
|
|
//
|
|
ClientSize = new System.Drawing.Size(384, 521);
|
|
Controls.Add(horizontalSeparator2);
|
|
Controls.Add(pickerBox1);
|
|
Controls.Add(button1);
|
|
Controls.Add(label2);
|
|
Controls.Add(label1);
|
|
Controls.Add(textBox1);
|
|
MinimumSize = new System.Drawing.Size(400, 560);
|
|
Name = "EditGroupForm";
|
|
Text = "Edit Group";
|
|
ResumeLayout(false);
|
|
PerformLayout();
|
|
}
|
|
|
|
public TileGroupModel Result
|
|
{
|
|
get
|
|
{
|
|
if (result == null)
|
|
{
|
|
result = new TileGroupModel();
|
|
}
|
|
|
|
result.Title = textBox1.Text?.Trim() ?? string.Empty;
|
|
result.IsExpanded = pickerBox1.Value;
|
|
|
|
return result;
|
|
}
|
|
}
|
|
|
|
}
|
|
} |